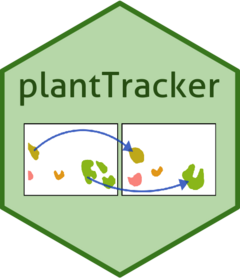
Calculates the number of recruits of each species per year in each quadrat
Source:R/getRecruits.R
getRecruits.Rd
This function calculates the number of new recruits of
each species in each quadrat in each year. The input data must already
contain a column indicating whether each observation is classified as a
recruit or not. This recruit status can be generated from the
trackSpp
function in plantTracker
, or can be information that
was collected in the field. This function includes an option that determines
whether each ramet of a clonal species is considered an individual recruit,
or if the entire genet is considered a single recruit.
Usage
getRecruits(
dat,
byGenet = TRUE,
species = "Species",
quad = "Quad",
site = "Site",
year = "Year",
trackID = "trackID",
recruit = "recruit",
...
)
Arguments
- dat
An sf data.frame in which each row represents a unique polygon (either a genet or a ramet) in a unique site/quadrat/year combination. A data.frame returned by
trackSpp
can be put directly into this function. 'dat' must have columns that contain...a unique identification for each research site in character format with no NAs (the default column name is "Site")
species name in character format with no NAs (the default column name is "Species")
unique quadrat identifier in character format with no NAs (the default column name is "Quad")
year of data collection in integer format with no NAs (the default column name is "Year")
a unique identifier for each genet in character format with no NAs (the default column name is "trackID")
an s.f 'geometry' column that contains a polygon or multipolygon data type for each individual observation (the default column name is "geometry")
- byGenet
A logical argument.
TRUE
indicates that a new genet will be considered as only one recruit, even if it consists of multiple ramets.FALSE
indicates that each new ramet will be considered as a new recruit, even if other ramets of the same genet were present in previous years.- species
An optional character string argument. Indicates the name of the column in 'dat' that contains species name data. It is unnecessary to include a value for this argument if the column name is "Species" (default value is 'Species').
- quad
An optional character string argument. Indicates the name of the column in 'dat' that contains quadrat name data. It is unnecessary to include a value for this argument if the column name is "Quad" (default is 'Quad').
- site
An optional character string argument. Indicates the name of the column in 'dat' that contains site name data. It is unnecessary to include a value for this argument if the column name is "Site" (default value is 'Site').
- year
An optional character string argument. Indicates the name of the column in 'dat' that contains data for year of sampling. It is unnecessary to include a value for this argument if the column name is "Year" (default is 'Year').
- trackID
An optional character string argument. Indicates the name of the column in 'dat' that contains unique identifiers for each genet. It is unnecessary to include a value for this argument if the column name is "trackID" (default is 'trackID')
- recruit
An optional character string argument. Indicates the name of the column in 'dat' that contains information indicating whether or not this row represents data for a recruit. It is unnecessary to include a value for this argument if the column name is "recruit" (default is "recruit").
- ...
Other arguments passed on to methods. Not currently used.
Value
This function returns a table with columns for site, quadrat, species name, year, and number of recruits.
This function returns a data frame with the columns 'Site', 'Quad', 'speciesName', 'Year', and 'recruits'. The 'recruits' column contains a count of the number of individuals of the species and in the site, quadrat, and year indicated in the other columns of the data frame.
Examples
dat <- grasslandData[grasslandData$Site == c("AZ") &
grasslandData$Species %in% c("Bouteloua rothrockii",
"Calliandra eriophylla") &
grasslandData$Year %in% c(1922:1925),]
names(dat)[1] <- "speciesName"
inv <- grasslandInventory[unique(dat$Quad)]
outDat <- trackSpp(dat = dat,
inv = inv,
dorm = 1,
buff = .05,
buffGenet = 0.005,
clonal = data.frame("Species" = unique(dat$speciesName),
"clonal" = c(TRUE,FALSE)),
species = "speciesName",
aggByGenet = TRUE
)
#> Site: AZ
#> -- Quadrat: SG2
#> ---- Species: Bouteloua rothrockii
#> ; Calliandra eriophylla
#> Note: Individuals in year 1927 have a value of 'NA' in the 'survives_tplus1' and 'size_tplus1' columns because 1927 is the last year of sampling in this quadrat.
#> -- Quadrat: SG4
#> ---- Species: Bouteloua rothrockii
#> ; Calliandra eriophylla
#> Note: Individuals in year 1927 have a value of 'NA' in the 'survives_tplus1' and 'size_tplus1' columns because 1927 is the last year of sampling in this quadrat.
#> Note: The output data.frame from this function is shorter than your input data.frame because demographic data has been aggregated by genet. Because of this, some columns that were present in your input data.frame may no longer be present. If you don't want the output to be aggregated by genet, include the argument 'aggByGenet == FALSE' in your call to trackSpp().
getRecruits(dat = outDat,
byGenet = TRUE,
species = "speciesName"
)
#> Site Quad speciesName Year recruits
#> 1 AZ SG2 Bouteloua rothrockii 1923 20
#> 2 AZ SG2 Bouteloua rothrockii 1924 5
#> 3 AZ SG2 Bouteloua rothrockii 1925 21
#> 4 AZ SG2 Calliandra eriophylla 1925 1
#> 5 AZ SG4 Bouteloua rothrockii 1923 19
#> 6 AZ SG4 Bouteloua rothrockii 1924 5
#> 7 AZ SG4 Bouteloua rothrockii 1925 8
#> 8 AZ SG4 Calliandra eriophylla 1924 3