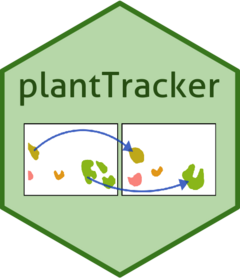
Calculates local neighborhood density around each individual in a mapped dataset
Source:R/getNeighbors.R
getNeighbors.Rd
This function calculates the density of individuals around
each distinct individual in a mapped dataset. It is intended for use on
a dataset that has been returned by the trackSpp
function, but
will work with any sf data.frame where each individual row represents a
distinct individual (genet) in a unique year.
Usage
getNeighbors(
dat,
buff,
method,
compType = "allSpp",
output = "summed",
trackID = "trackID",
species = "Species",
quad = "Quad",
year = "Year",
site = "Site",
geometry = "geometry",
...
)
Arguments
- dat
An sf data.frame. Each row must represent a unique individual organism in a unique year. This argument can be a data.frame that is returned by the
trackSpp
function. It must have columns that contain... #' * a unique identification for each research site in character format with no NAs (the default column name is "Site")species name in character format with no NAs (the default column name is "Species")
unique quadrat identifier in character format with no NAs (the default column name is "Quad")
year of data collection in integer format with no NAs (the default column name is "Year")
a unique identifier for each genet in character format with no NAs (the default column name is "trackID")
an s.f 'geometry' column that contains a polygon or multipolygon data type for each individual observation (the default column name is "geometry")
- buff
A numeric value that is greater than or equal to zero. This indicates the distance (in the same units as the spatial data in 'dat') around each focal individual within which you want to look for competitors. OR buff can be a data.frame with the columns "Species" and "buff". This data.frame must have a row for each unique species present in 'dat', with species name as a character string in the "Species" column, and a numeric value in the 'buff' column you'd like to use for that species.
- method
A character string, either 'count' or 'area'. This argument determines which method is used to calculate local neighborhood density. If 'method' = 'count', then the number of other individuals within the buffer will be returned in the 'neighbors' column. If method = 'area', then the proportion of the buffer area that is occupied by other individuals will be returned in the 'neighbors' column. If the data in 'dat' was mapped initially as points, it is best to use 'method' = 'count'. If the data was mapped as polygons that are representative of individual basal area, using 'method' = 'area' is likely a more accurate representation of the crowding the focal individual is experiencing.
- compType
A character string, either 'allSpp' or 'oneSpp'. If compType = 'allSpp', then local neighborhood density is calculated considering all individuals around the focal individual, regardless of species (interspecific competition). If compType = 'oneSpp', then local neighborhood density is calculated considering only individuals in the buffer area that are the same species as the focal individual (intraspecific competition).
- output
A character string, either 'summed' or 'bySpecies'. The default is 'summed'. This argument is only important to consider if you are using compType = 'allSpp'. If output = 'summed', then only one count/area value is returned for each individual. This value is the total count or area of all neighbors within the focal species buffer zone, regardless of species. If output = 'bySpecies', there is a count or area value returned for each species present in the buffer zone. For example, there are 15 individuals inside a buffer zone. Five are species A, three are species B, and 7 are species C. If output = 'summed', then the 'neighbors_count' column in the output data.frame will have the single value '15' in the row for this focal individual. However, if output = 'bySpecies', the row for this focal individual in the output data.frame will contain a named list in the 'neighbors_count' column that looks like the one below. If 'method' = 'area' and 'output' = 'bySpecies', a similar list will be returned, but will be in the 'neighbors_area' column and will contain areas rather than counts.
list("Species A "= 5, "Species B" = 3, "Species C" = 7) #> $`Species A ` #> [1] 5 #> #> $`Species B` #> [1] 3 #> #> $`Species C` #> [1] 7
- trackID
An optional character string argument. Indicates the name of the column in 'dat' that contains a value that uniquely identifies each individual/genet. It is unnecessary to include a value for this argument if the column name is 'trackID' (the default value is 'trackID').
- species
An optional character string argument. Indicates the name of the column in 'dat' that contains species name data. It is unnecessary to include a value for this argument if the column name is "Species" (default value is 'Species').
- quad
An optional character string argument. Indicates the name of the column in 'dat' that contains quadrat name data. It is unnecessary to include a value for this argument if the column name is "Quad" (default is 'Quad').
- year
An optional character string argument. Indicates the name of the column in 'dat' that contains data for year of sampling. It is unnecessary to include a value for this argument if the column name is "Year" (default is 'Year').
- site
An optional character string argument. Indicates the name of the column in 'dat' that contains site name data. It is unnecessary to include a value for this argument if the column name is "Site" (default value is 'Site').
- geometry
An optional character string argument. Indicates the name of the column in 'dat' that contains sf geometry data. It is unnecessary to include a value for this argument if the column name is "geometry" (default is 'geometry').
- ...
Other arguments passed on to methods. Not currently used.
Value
This function returns a data.frame with the same number of rows as 'dat' and all of the same columns, but with an additional column or columns. If method = 'count', then a column called "neighbors_count" is added, which contains a count of the number of individuals within the buffer area that is occupied by other individuals. If method = 'area', two columns are added. The first is called "nBuff_area", which contains the area of the buffer around the focal individual. The second is called "neighbors_area", which contains the area of the individuals within the buffer zone around the focal individual.
Details
This function draws a buffer around each individual genet of a distance specified by the user. Then it either counts the number of other genets within that buffer, or calculates the proportion of that buffer area that is occupied by other individuals. getNeighbors can calculate either interspecific local neighborhood density (between the focal individual and all other individuals within the buffer area) or intraspecific local neighborhood density (between the focal individual and other individuals of the same species within the buffer area).
See also
The trackSpp()
function returns a data.frame that can be input
directly into this function. If a data.frame is not aggregated by genet such
that each unique genet/year combination is represented by only one row, then
passing it through the aggregateByGenet()
function will return a data.frame
that can be input directly into this function.
Examples
dat <- grasslandData[grasslandData$Site == c("AZ") &
grasslandData$Species %in% c("Bouteloua rothrockii",
"Calliandra eriophylla"),]
names(dat)[1] <- "speciesName"
inv <- grasslandInventory[unique(dat$Quad)]
outDat <- trackSpp(dat = dat,
inv = inv,
dorm = 1,
buff = .05,
buffGenet = 0.005,
clonal = data.frame("Species" = unique(dat$speciesName),
"clonal" = c(TRUE)),
species = "speciesName",
aggByGenet = TRUE
)
#> Site: AZ
#> -- Quadrat: SG2
#> ---- Species: Bouteloua rothrockii
#> ; Calliandra eriophylla
#> Note: Individuals in year 1927 have a value of 'NA' in the 'survives_tplus1' and 'size_tplus1' columns because 1927 is the last year of sampling in this quadrat.
#> -- Quadrat: SG4
#> ---- Species: Bouteloua rothrockii
#> ; Calliandra eriophylla
#> Note: Individuals in year 1927 have a value of 'NA' in the 'survives_tplus1' and 'size_tplus1' columns because 1927 is the last year of sampling in this quadrat.
#> Note: The output data.frame from this function is shorter than your input data.frame because demographic data has been aggregated by genet. Because of this, some columns that were present in your input data.frame may no longer be present. If you don't want the output to be aggregated by genet, include the argument 'aggByGenet == FALSE' in your call to trackSpp().
finalDat <- getNeighbors(dat = outDat,
buff = .15,
method = 'count',
compType = 'oneSpp',
species = "speciesName")