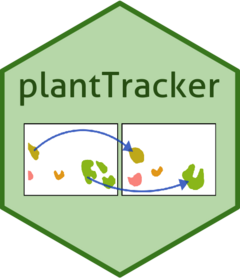
Calculates lambda, the population growth rate, for each species in a quadrat based on changes in basal cover.
Source:R/getLambda.R
getLambda.Rd
This function calculates the population growth rate (lambda) for
every species in a quadrat. This value is the ratio of basal area or number
of individuals in the next year to basal area or number of individuals in the
current year (basal area in year t+1/ basal area in year t). A lambda value
greater than 1 indicates a population is growing, while a value less than 1
indicates population decline. Lambda is 'infinity' when the basal area or
number of individuals in year t is 0, and is NA when basal area or number of
individuals in year t is zero (i.e. when there are no organims present in
year =t). Note that a lambda value is calculated between of the years when a
quadrat was sampled, even if there is a gap in sampling. For example, a
quadrat is sampled in 1998, 1999, 2001, and 2002 (but skipped in 2000). A
lambda value will be calculated for 1998-1999 and 2001-2002, which is a
transition from year t
to year t+1
. However, a lambda value is calculated
in the same manner for 1999-2001, which is actually a transition from year
t
to year t+2
. You can remove these values by subsetting the data.frame
returned by getLambda()
for rows when "Year_tplus1"- "Year_t" is equal
to 1.
Usage
getLambda(
dat,
inv,
method = "area",
species = "Species",
quad = "Quad",
site = "Site",
year = "Year",
geometry = "geometry",
...
)
Arguments
- dat
An sf data.frame in which each row represents a unique polygon (either a genet or a ramet) in a unique site/quadrat/year combination. A data.frame returned by
trackSpp
can be put directly into this function. However, it is not strictly necessary for 'dat' to have demographic data or unique identifiers (i.e. 'trackIDs') assigned. If there are not trackIDs assigned for each individual, then the function assumes that each row of data repesents one genetic individual.'dat' must have columns that contain...a unique identification for each research site in character format with no NAs (the default column name is "Site")
species name in character format with no NAs (the default column name is "Species")
unique quadrat identifier in character format with no NAs (the default column name is "Quad")
year of data collection in integer format with no NAs (the default column name is "Year")
an s.f 'geometry' column that contains a polygon or multipolygon data type for each individual observation (the default column name is "geometry")
- inv
The name of each element of the list is a quadrat name in 'dat', and the contents of that list element is a numeric vector of all of the years in which that quadrat (or other unique spatial area) was sampled. Make sure this is the years the quadrat was actually sampled, not just the years that have data in the 'dat' argument! This argument allows the function to differentiate between years when the quadrat wasn't sampled and years when there just weren't any individuals of a species present in that quadrat.
- method
A single character argument that determines the method for calculating lambda. The default value is "area", which means that lambda is calculated by comparing total basal area for a given species in year t+1 to year t. If 'method' = "count", then lambda is calculated by comparing total number of individuals for a given species in year t+1 to year t. If each individual in 'dat' is mapped as a point, it is best to use 'method' = "count".
- species
An optional character string argument. Indicates the name of the column in 'dat' that contains species name data. It is unnecessary to include a value for this argument if the column name is "Species" (default value is 'Species').
- quad
An optional character string argument. Indicates the name of the column in 'dat' that contains quadrat name data. It is unnecessary to include a value for this argument if the column name is "Quad" (default is 'Quad').
- site
An optional character string argument. Indicates the name of the column in 'dat' that contains site name data. It is unnecessary to include a value for this argument if the column name is "Site" (default value is 'Site').
- year
An optional character string argument. Indicates the name of the column in 'dat' that contains data for year of sampling. It is unnecessary to include a value for this argument if the column name is "Year" (default is 'Year').
- geometry
An optional character string argument. Indicates the name of the column in 'dat' that contains sf geometry data. It is unnecessary to include a value for this argument if the column name is "geometry" (default is 'geometry').
- ...
Other arguments passed on to methods. Not currently used.
Value
This function returns a data.frame with columns containing site, quadrat, and species data, as well as the following columns:
- Year_t
the 'current' year
- absolute_basalArea_t
basal area (in the same units as the spatial elements of 'dat') for this species in this quadrat in year 't'
- Year_tplus1
the 'next' year
- absolute_basalArea_tplus1
basal area (in the same units as the spatial elements of 'dat') for this species in this quadrat in year 't+1'
- lambda
The population growth rate for this species in this quadrat from year t to year t+1.
See also
getBasalAreas()
, used internally in this function, which
calculates absolute and relative basal areas for each species in each quadrat
for each year of sampling.
Examples
dat <- grasslandData[grasslandData$Site == "AZ" &
grasslandData$Year %in% c(1922:1925),]
names(dat)[1] <- "speciesName"
inv <- grasslandInventory[unique(dat$Quad)]
outDat <- trackSpp(dat = dat,
inv = inv,
dorm = 1,
buff = .05,
buffGenet = 0.005,
clonal = data.frame("Species" = unique(dat$speciesName),
"clonal" = c(TRUE,FALSE)),
species = "speciesName",
aggByGenet = TRUE
)
#> Site: AZ
#> -- Quadrat: SG2
#> ---- Species: Heteropogon contortus
#> ; Bouteloua rothrockii
#> ; Ambrosia artemisiifolia
#> ; Calliandra eriophylla
#> Note: Individuals in year 1927 have a value of 'NA' in the 'survives_tplus1' and 'size_tplus1' columns because 1927 is the last year of sampling in this quadrat.
#> -- Quadrat: SG4
#> ---- Species: Bouteloua rothrockii
#> ; Ambrosia artemisiifolia
#> ; Calliandra eriophylla
#> Note: Individuals in year 1927 have a value of 'NA' in the 'survives_tplus1' and 'size_tplus1' columns because 1927 is the last year of sampling in this quadrat.
#> Note: The output data.frame from this function is shorter than your input data.frame because demographic data has been aggregated by genet. Because of this, some columns that were present in your input data.frame may no longer be present. If you don't want the output to be aggregated by genet, include the argument 'aggByGenet == FALSE' in your call to trackSpp().
getLambda(dat = outDat, inv = inv, method = "area",
species = "speciesName")
#> Site Quad speciesName Year_t absolute_basalArea_t Year_tplus1
#> 1 AZ SG2 Ambrosia artemisiifolia 1922 8.862780e-04 1923
#> 2 AZ SG2 Ambrosia artemisiifolia 1923 0.000000e+00 1924
#> 3 AZ SG2 Ambrosia artemisiifolia 1924 0.000000e+00 1925
#> 4 AZ SG2 Ambrosia artemisiifolia 1925 0.000000e+00 1926
#> 5 AZ SG2 Ambrosia artemisiifolia 1926 0.000000e+00 1927
#> 6 AZ SG2 Heteropogon contortus 1922 2.820466e-02 1923
#> 7 AZ SG2 Heteropogon contortus 1923 3.071770e-02 1924
#> 8 AZ SG2 Heteropogon contortus 1924 2.985456e-02 1925
#> 9 AZ SG2 Heteropogon contortus 1925 7.084470e-03 1926
#> 10 AZ SG2 Heteropogon contortus 1926 0.000000e+00 1927
#> 11 AZ SG2 Bouteloua rothrockii 1922 7.655839e-03 1923
#> 12 AZ SG2 Bouteloua rothrockii 1923 4.225758e-03 1924
#> 13 AZ SG2 Bouteloua rothrockii 1924 4.278202e-03 1925
#> 14 AZ SG2 Bouteloua rothrockii 1925 7.640600e-03 1926
#> 15 AZ SG2 Bouteloua rothrockii 1926 0.000000e+00 1927
#> 16 AZ SG2 Calliandra eriophylla 1922 4.923767e-05 1923
#> 17 AZ SG2 Calliandra eriophylla 1923 0.000000e+00 1924
#> 18 AZ SG2 Calliandra eriophylla 1924 4.923767e-05 1925
#> 19 AZ SG2 Calliandra eriophylla 1925 7.385650e-05 1926
#> 20 AZ SG2 Calliandra eriophylla 1926 0.000000e+00 1927
#> 21 AZ SG4 Ambrosia artemisiifolia 1922 2.461883e-04 1923
#> 22 AZ SG4 Ambrosia artemisiifolia 1923 0.000000e+00 1924
#> 23 AZ SG4 Ambrosia artemisiifolia 1924 0.000000e+00 1925
#> 24 AZ SG4 Ambrosia artemisiifolia 1925 0.000000e+00 1926
#> 25 AZ SG4 Ambrosia artemisiifolia 1926 0.000000e+00 1927
#> 26 AZ SG4 Bouteloua rothrockii 1922 2.680527e-03 1923
#> 27 AZ SG4 Bouteloua rothrockii 1923 2.782187e-03 1924
#> 28 AZ SG4 Bouteloua rothrockii 1924 2.404456e-03 1925
#> 29 AZ SG4 Bouteloua rothrockii 1925 2.199422e-03 1926
#> 30 AZ SG4 Bouteloua rothrockii 1926 0.000000e+00 1927
#> 31 AZ SG4 Calliandra eriophylla 1922 0.000000e+00 1923
#> 32 AZ SG4 Calliandra eriophylla 1923 0.000000e+00 1924
#> 33 AZ SG4 Calliandra eriophylla 1924 7.385650e-05 1925
#> 34 AZ SG4 Calliandra eriophylla 1925 0.000000e+00 1926
#> 35 AZ SG4 Calliandra eriophylla 1926 0.000000e+00 1927
#> absolute_basalArea_tplus1 lambda
#> 1 0.000000e+00 0.0000000
#> 2 0.000000e+00 NaN
#> 3 0.000000e+00 NaN
#> 4 0.000000e+00 NaN
#> 5 0.000000e+00 NaN
#> 6 3.071770e-02 1.0891004
#> 7 2.985456e-02 0.9719008
#> 8 7.084470e-03 0.2372994
#> 9 0.000000e+00 0.0000000
#> 10 0.000000e+00 NaN
#> 11 4.225758e-03 0.5519654
#> 12 4.278202e-03 1.0124106
#> 13 7.640600e-03 1.7859372
#> 14 0.000000e+00 0.0000000
#> 15 0.000000e+00 NaN
#> 16 0.000000e+00 0.0000000
#> 17 4.923767e-05 Inf
#> 18 7.385650e-05 1.5000000
#> 19 0.000000e+00 0.0000000
#> 20 0.000000e+00 NaN
#> 21 0.000000e+00 0.0000000
#> 22 0.000000e+00 NaN
#> 23 0.000000e+00 NaN
#> 24 0.000000e+00 NaN
#> 25 0.000000e+00 NaN
#> 26 2.782187e-03 1.0379256
#> 27 2.404456e-03 0.8642321
#> 28 2.199422e-03 0.9147275
#> 29 0.000000e+00 0.0000000
#> 30 0.000000e+00 NaN
#> 31 0.000000e+00 NaN
#> 32 7.385650e-05 Inf
#> 33 0.000000e+00 0.0000000
#> 34 0.000000e+00 NaN
#> 35 0.000000e+00 NaN